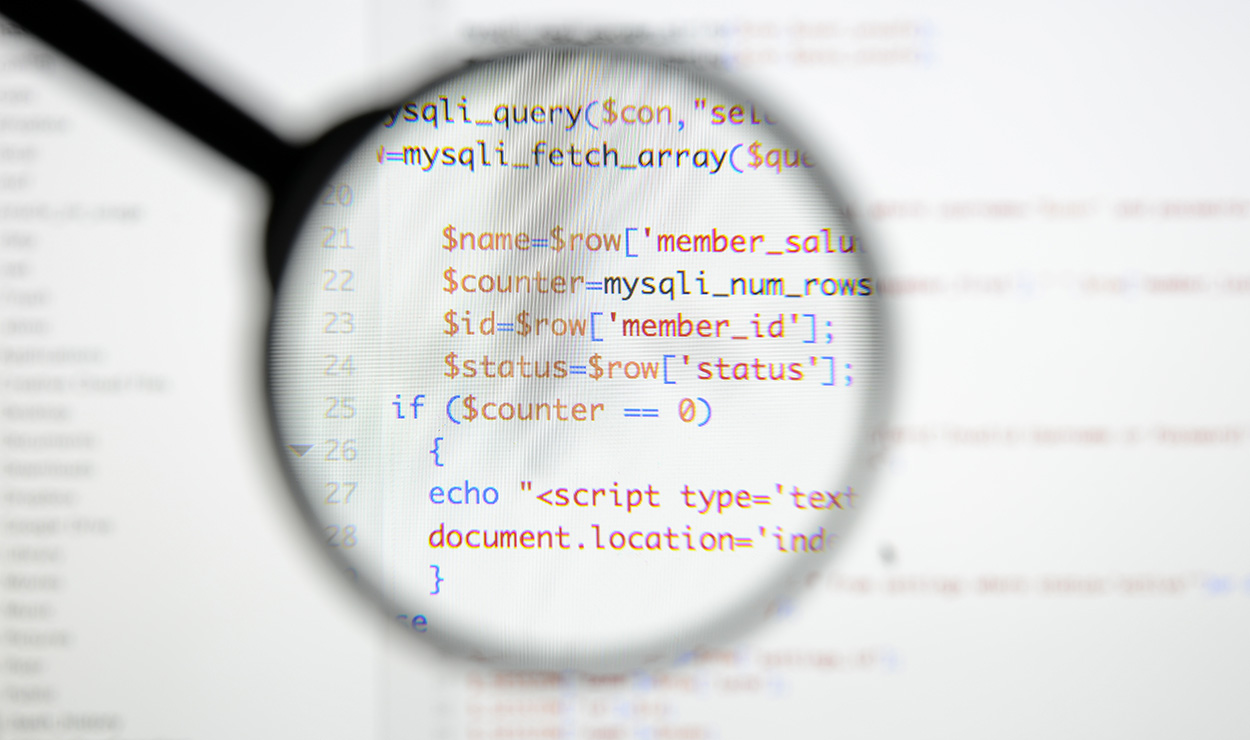
Code review is an integral part of the development cycle, with a direct impact on quality. Whether you’re focused on web, plugin, or theme development, thorough code review can help you improve your final product. In this article, we look at the pros and cons of code review in WordPress, some of the most common mistakes to watch for in PHP, JavaScript, HTML, and tips to make sure your code reviews are worth the investment in time and energy.
Pros and Cons of WordPress Code Review
There are plenty of benefits to code review:
Quality Assurance: The primary aim of code review is to ensure the code follows WordPress standards, is functional, and secure. This makes it less likely that bugs or errors will appear in the final product.
Improved Security: The popularity of WordPress makes it a target, so maintaining security is paramount. Code review helps identify possible vulnerabilities early, such as unsanitized inputs or potential SQL injections.
Consistency: Code review helps ensure consistency, especially in larger projects with many contributors. Consistency makes code easier to read and understand.
Learning Opportunities: Code reviews should be a learning experience. Feedback helps developers write code that’s cleaner, more secure, and efficient.
On the downside, code review is time consuming, requires highly-experienced developers, and can lead to interpersonal conflicts, especially in situations where code quality might be subjective or when personal coding styles differ significantly.
Code reviews are also limited in scope. Despite being a critical process, code review can’t catch all types of bugs and vulnerabilities. Complementary practices such as unit testing, integration testing, and static analysis can help contribute to robust code.
Make Your Code Reviews the Best They Can Be
Your code review is only as good as your reviewers and your process. Below are five of the most common mistakes when it comes to reviewing WordPress code. These are general errors in review technique, rather than specific errors you’ll need to watch for in PHP, JavaScript, and HTML.
Ignoring Coding Standards
WordPress has defined a set of coding standards to ensure a certain degree of coding quality and consistency across different projects. A particular project may have reason to depart from these standards in some way, but that should be thoroughly documented and used consistently. When it comes to code review, it’s vital to possess a thorough understanding of the coding standards being used.
Code that doesn’t follow a set of standards can be inconsistent and difficult to understand or maintain. Part of the reviewer’s job is to prevent this from happening.
Tools like Code Sniffer, equipped with WordPress Coding Standards, can help identify coding standard discrepancies.
Coding Standards vs. Coding Style
“Coding style” and “coding standards” are very different things.
Coding style largely refers to the cosmetic aspects of your code. It’s at least somewhat subjective and can vary from one developer to the next.
However, when you’re part of a team or contributing to open-source projects like WordPress, a consistent coding style makes code easier to read and comprehend. This reduces the cognitive load for everyone working on the project, including in the review stage.
A coding standard is more objective, and enforces certain rules to ensure the code’s reliability, efficiency, and maintainability. Adherence to WordPress coding standards helps ensure that the code is secure, efficient, and works cohesively within the WordPress ecosystem.
Skipping Reviews
In the haste to get the code into production, developers may skip the code review process altogether. Very often, this is because the changes are relatively small. However, even “minor” bugs being pushed into the production code can have severe ramifications.
Ignoring the UX
This is more of a philosophical question. The code itself may be rock solid, but how does it affect the user’s experience? If UX changes for the better (or at least stays the same), then you have no problem. Changes that will negatively impact the user experience need more careful consideration. Watch out for errors such as obstructive notifications, or updates that should instead be managed on the plugin settings page.
The knee jerk reaction may be to simply reject anything that makes the user experience worse, but you need to have a holistic, long-term view. The patch you put out today might make UX slightly worse in the near future, but it may be worth it if it helps create a better user experience in the future.
Lacking a Broad View
Don’t ignore the impact of code changes on other parts of the system. Changes to a single function could potentially break other parts of the software if not properly reviewed.
Namespace and prefix clashes may be the most common cause of this in WordPress. The problem is usually easy to solve, but you’ve got to spot it first.
Understanding the full impact of code changes can help prevent unintentional side effects. Run complete system tests in a development environment to ensure no integral parts of the system are disrupted by these changes.
Trusting Your Colleagues Too Much
“Surely (COWORKER) knows what they’re doing. They’ve been coding for 25 years!”
This attitude is the death knell for any attempt at a thorough code review. Everyone makes mistakes sometimes. There are no exceptions to this rule. No doubt your colleagues do know exactly what they’re doing…
…and they’re never sleepy, never hungry, never distracted by personal problems, and always 100% focused on the task at hand.
Never assume someone has done their job correctly when you’re reviewing their work. More experience than you? Doesn’t matter. It’s your boss’s code? Doesn’t matter. They’ve literally won awards for their sparkling clean code, given several TED talks on the subject, and hold a PhD in Computer Science? Does. Not. Matter!
Your job is to review. Keep your attention focused on what’s in front of you, not the bona fides of the person who wrote it.
Common Code Errors
Some mistakes are more common than others. Below we’ve assembled some of the most common errors in PHP, JavaScript, and HTML. In all cases, the code you write should also follow the Accessibility Coding Standards.
Common Mistakes in PHP
Data Validation
Failing to validate, sanitize, or escape data can lead to security vulnerabilities. Solid code review can help prevent this, and ensure the right processes are being followed.
Data validation involves checking if the data meets the expected format. WordPress offers several functions to validate data, such as is_email() for email validation.
$email = '[email protected]';
if ( is_email($email) ) {
echo 'This is a valid email address.' ;
} else {
echo 'Invalid email address.' ;
}
Data sanitization is the process of cleaning or filtering your input data. Sanitization processes depend on the type of data you’re working with. WordPress provides multiple sanitization functions, such as sanitize_text_field()
, sanitize_email()
, and sanitize_url()
.
$dirty_text = ' Hello world! < /br > ';
$clean_text = sanitize_text_field($dirty_text);
echo $clean_text; // Outputs: "Hello world!"
Data escaping involves securing output data displayed to the user. This ensures malicious scripts are not executed in the user’s browser. The exact WordPress escaping functions vary depending on the type of data being escaped.
Naming Conventions
During your review, make sure all PHP variables and function names are formatted according to the WordPress naming conventions.
Functions, Actions, Filters, and Variables
These should always be in lowercase, with words separated by underscores rather than spaces.
// Example follows PHP naming conventions
function my_custom_function() {
// Code goes here
}
// Example does not follow PHP naming conventions
function MyCustomFunction() {
// Code goes here
}
Classes
Everything said about classes also applies to traits, interfaces, and enums. For class names in WordPress, each word should start with a capital letter, with an underscore between each word. Acronyms should be in all capitals.
Constants
Constants should be all uppercase, with words separated by underscores.
define( 'MY_CUSTOM_CONSTANT' , true );
File Names
WordPress PHP file names should be lowercase and words should be separated by hyphens, not underscores. However, this isn’t the only thing to watch for when reviewing. Make sure every file has a descriptive name. Someone should be able to hazard a guess at what any file does from the name alone. This may not always be possible, but it’s a goal to aim for.
Class file names follow the same conventions as other file names, but based on the class name. Prepend class-
to the class name, and replace underscores with hyphens.
Indentation
Indentation refers to the space at the beginning of each line of code. Good indentation provides a clear hierarchy, showing how blocks of code are related to each other.
When reviewing, you’re mostly checking to see if indentation is used consistently throughout, and to make sure that each nested statement is indented once more than the statement before.
Common Mistakes in JavaScript
If PHP is the backbone of WordPress, then JavaScript is some…other…important body part. The brain, maybe?
Here are some common mistakes to watch out for when reviewing WordPress JavaScript:
Improper Naming Conventions: In JavaScript, variables and function names should be camelCase, not underscore-separated as in PHP. Mixing up these conventions is a frequent mistake.
Using
==
Instead of===
: Using the==
operator can cause unexpected type coercion. It’s standard practice in WordPress to use the===
operator for equality comparisons, to ensure both value and type match.Improperly Declared Variables: Variables in JavaScript are declared with
var
,let
, orconst
, each with unique properties and use cases.
var
: This is the oldest way to declare variables in JavaScript. Use ofvar
is generally discouraged these days in favor oflet
andconst
. Variables declared withvar
are function-scoped, hoisted to the top of the function, and can be updated and re-declared in the scope. A variable declared withvar
can be declared without initialization.let
: Variables declared withlet
are block-scoped. They are hoisted, but not initialized. Variables can be updated but not redeclared. They can be declared without initialization, but accessing them without it will give aReferenceError
.const
: This is used to declare constants. Likelet
, it is block-scoped and hoisted, but not initialized. Constants cannot be updated or redeclared in the scope. Variables usingconst
can’t be declared or accessed without initialization.
Common Mistakes in HTML
Improper use of HTML tags: Check to make sure all tags have been used according to their semantic meaning, not their appearance. For example, misuse of heading tags can lead to poor SEO optimization and hamper accessibility.
Missing closing tags: This is an error commonly made by novices rather than experienced developers, but that’s part of the danger. It’s easy to miss just one of these, and it can be hard to spot if you’re only doing a cursory review.
Ignoring Accessibility: HTML will run just fine if the
alt
attribute is left out of<img>
tags, but you need to remember that some people depend on alt text to help them make sense of images. During your review, take a moment to make sure that thealt
attribute is present and actually provides some value. The name of the file, or a vague description of what’s going on, such as “Screenshot of plugin in action,” isn’t going to cut it. There’s a lot more to Accessibility Standards, but missing or pointless alt text is a very common problem.Invalid Nesting: Make sure all the HTML tags are properly nested. Improper nesting can create rendering issues in browsers. Just like leaving out a closing tag, this error is more associated with neophytes than seasoned professionals. Again, this can make them tough to spot if you’re not reviewing with your full attention. You don’t expect the developer to make that mistake, so you don’t look for it.
Wrapping Up
Code reviews are not just checking boxes in the development process, but a way to ensure the longevity and reliability of your code. By adhering to WordPress coding standards, mandatory review processes, focusing on UX, and minding the impact of code changes, you can avoid standard code review mistakes.
How is your code review process? If you’re a freelancer, what steps do you take to make sure your code is robust? Let us know in the comments.