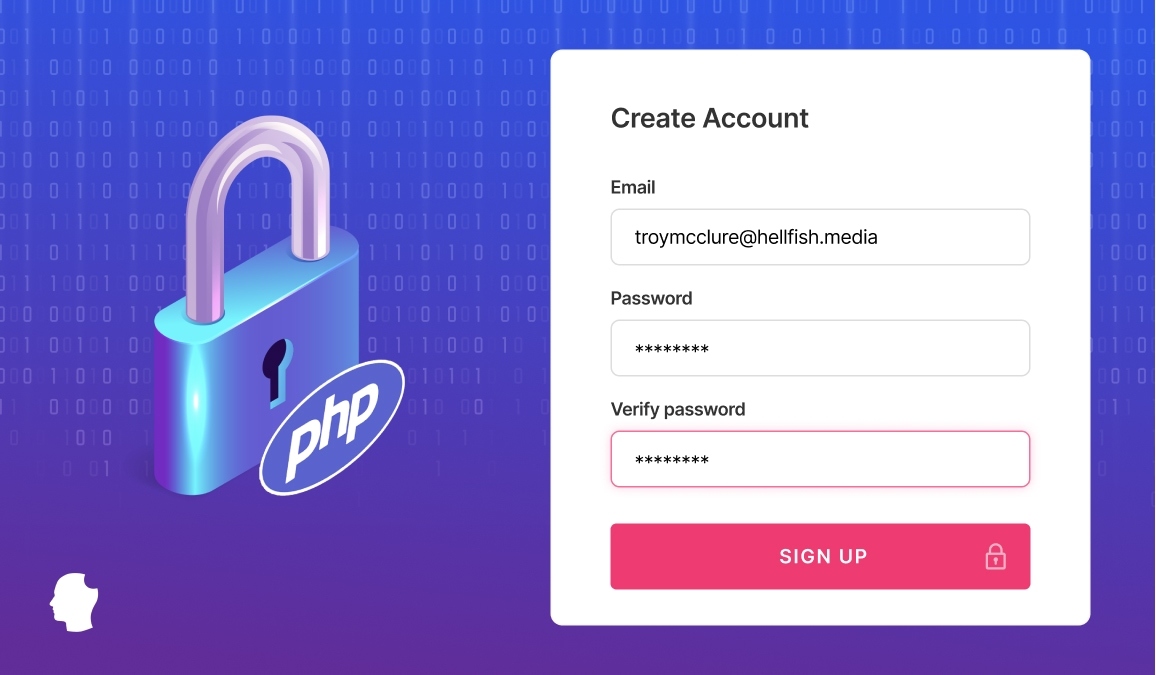
A few years ago I attended Laracon EU where Marcus Bointon gave a great talk on Crypto in PHP 7.2. I left the talk having a much greater appreciation for how vastly complicated cryptography is, but also for how PHP is making encryption more accessible thanks to the introduction of Sodium. Data encryption in PHP has been vital to my work on SpinupWP, a cloud-based server control panel with root access to thousands of servers and sites, and I thought it would be useful to share what I’ve learned since then. Buckle up, because this could be a bumpy ride!
Types of Encryption
There are a range of different encryption methods in use today, the most common being hashing, secret key encryption, and envelope encryption. In addition, each encryption method has multiple algorithms or ciphers to choose from (each with its own strengths and weaknesses). In this article, we’re going to look at implementing all three encryption methods.
Hashing
A hashing algorithm takes an input value and transforms it to a fixed-length output known as a “message digest”, “hash value” or simply a “hash”. Hashing is one way only, which means that the only way to validate a hashed output is to pass the original value to the hashing algorithm and compare the results. This makes hashing perfect for storing user passwords.
It’s worth noting that hashing isn’t a bulletproof solution and not all hashing algorithms are equal. Consider MD5 and SHA1 which are fast and efficient, making them ideal for checksumming and file verification. However, their speed makes them unsuitable for hashing a user’s password.
With today’s computational power of modern CPUs/GPUs and cloud computing, a hashed password can be cracked by brute force in a matter of minutes. It’s fairly trivial to quickly generate billions of MD5 hashes from random words until a match is found, thereby revealing the original plaintext password. Instead, intentionally slower hashing algorithms such as bcrypt or Argon2 should be used.
While a hashed password generated by any algorithm will certainly obscure the original data and slow down any would-be attacker, we as developers should strive to use the strongest algorithm available. Luckily, PHP makes this easy thanks to password_hash()
.
$hash = password_hash($password, PASSWORD_DEFAULT);
The password_hash()
function not only uses a secure one-way hashing algorithm, but it automatically handles salt and prevents time based side-channel attacks. The function accepts a $password
to be encrypted, and a $algo
hashing algorithm. As of PHP 5.5, bcrypt (PASSWORD_BCRYPT
), which is based on the Blowfish cipher, will be used as the default hashing algorithm. Later versions of PHP introduced the Argon2 (PHP 7.2) and Argon2id (PHP 7.3) algorithms, but bcrypt is still the default. In fact, besides improvements to the password_hash()
function, the available options are considered so secure that no new algorithms have been introduced to the language for either PHP 8.0 or PHP 8.1.
It is possible to use Argon2 or Argon2id, if your version of PHP has been compiled with Argon2 support, by passing either the PASSWORD_ARGON2I
or PASSWORD_ARGON2ID
constant as the $algo
argument of the password_hash()
function. To check which algorithms are supported on your web host, you can launch the PHP interactive mode from the server command line, and run the password_algos
function. Below is an example of the output of that command on a standard SpinupWP server, where all three options are available.
[email protected]:~$ php -a
Interactive mode enabled
php > print_r(password_algos());
Array
(
[0] => 2y
[1] => argon2i
[2] => argon2id
)
php >
Verifying a user’s password is also a trivial process thanks to the password_verify()
function. Simply pass the plaintext password supplied by the user and compare it to the stored hash, like so:
if (password_verify($password, $hash)) {
echo "Let me in, I'm genuine!";
}
Notice how the password verification is performed in PHP. If you’re storing a user’s credentials in a database you may be inclined to hash the password entered at login and then perform a database SQL query, like so:
SELECT * FROM users
WHERE username = 'Ashley'
AND password = 'password_hash'
LIMIT 1;
This approach is susceptible to side-channel attacks and should be avoided. Instead, return the user and then check the password hash in PHP.
SELECT username, password FROM users
WHERE username = 'Ashley'
LIMIT 1;
if (password_verify($user->password, $hash)) {
echo "Let me in, I'm genuine!";
}
While hashing is great to encrypt passwords, it doesn’t work for arbitrary data that our application needs to access without user intervention. Let’s consider a billing application, which encrypts a user’s credit card information, typically saved from an HTML form. (Given the related legal and PCI compliance requirements, we don’t recommend building your own billing application, rather use something like Stripe. We’re just using this as an example). Each month our application needs to bill the user for their previous month’s usage. Hashing the credit card data wouldn’t work because it requires that our application be able to decrypt the hashed data which, as we pointed out earlier, is not possible with hashing.
Secret key encryption to the rescue!
Secret Key Encryption
Secret key encryption (or symmetric encryption as it’s also known) uses a single key to both encrypt and decrypt data. In the past PHP relied on mcrypt and openssl for secret key encryption. PHP 7.2 introduced Sodium, which is more modern and widely considered more secure. If you’re running an older version of PHP you can install Sodium via The PHP Extension Community Library aka PECL.
In order to encrypt a value, first you’ll need an encryption key, which can be generated using the sodium_crypto_secretbox_keygen()
function.
$key = sodium_crypto_secretbox_keygen();
You can also use the random_bytes()
function with the SODIUM_CRYPTO_SECRETBOX_KEYBYTES
integer constant for the key length, but using sodium_crypto_secretbox_keygen()
ensures that the key length is always correct (i.e. not too short), and it’s easier.
$key = random_bytes( SODIUM_CRYPTO_SECRETBOX_KEYBYTES );
Either way, you’ll usually only do this once and store the result as an environment variable. Remember that this key must be kept secret at all costs. If the key is ever compromised, so is any data encrypted by using it.
To encrypt the original value, pass it to the sodium_crypto_secretbox()
function with the $key
and a generated $nonce
. To create the nonce use the random_bytes()
function, with the SODIUM_CRYPTO_SECRETBOX_NONCEBYTES
integer constant for the nonce length, because the same nonce should never be reused.
$nonce = random_bytes( SODIUM_CRYPTO_SECRETBOX_NONCEBYTES );
$encrypted_result = sodium_crypto_secretbox( 'This is a secret!', $nonce, $key );
This presents a problem because we need the nonce to decrypt the value later. Luckily, nonces don’t have to be kept secret so we can prepend it to our $encrypted_result
then base64_encode()
the value before saving it to the database.
$encoded = base64_encode( $nonce . $encrypted_result );
This will create a base64 encoded string of 76 characters in length.
When it comes to decrypting the value, do the opposite, starting with decoding the base64 encoded string.
$decoded = base64_decode($encoded);
Because we know the length of the nonce (SODIUM_CRYPTO_SECRETBOX_NONCEBYTES
) we can extract it using mb_substr()
before decrypting the value.
$nonce = mb_substr($decoded, 0, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, '8bit');
$encrypted_result = mb_substr($decoded, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, null, '8bit');
$plaintext = sodium_crypto_secretbox_open($encrypted_result, $nonce, $key);
// string(17) "This is a secret!"
That’s all there is to secret key encryption in PHP, thanks to Sodium!
Envelope Encryption
While the approach outlined above is certainly a step in the right direction, it still leaves our data vulnerable if the secret key is compromised. Let’s consider a malicious user that gains access to the server that hosts our application. They won’t hang around on the server to decrypt any sensitive data. Rather, they’ll make a copy of the data and all the relevant files onto their own infrastructure and work to discover our secret key which we used to encrypt the data. This leaves our data completely exposed.
The simple solution is to not store our secret key in the same location as the encrypted data, but this presents a problem. How do we encrypt and decrypt on demand? Enter Key Management Services (KMS) which allow you to securely host keys on their platform. Companies that offer cloud infrastructure like AWS and Google each have their own offerings. As a quick tutorial, we’ll be focusing on Google’s Cloud KMS.
Google Cloud KMS
Cloud KMS is a service provided by Google for securely hosting cryptographic keys. It provides a variety of useful features around key storage, including automatic key rotation and delayed key destruction. However, we’re primarily concerned with storing our secret key separately from our application.
To make things more secure we’re going to use a technique known as envelope encryption. Essentially, envelope encryption involves encrypting keys with another key. We do this for two reasons:
- Cloud KMS has a size limit of 64 KiB on the data that can be encrypted and decrypted. Therefore, it may not be possible to send all of the data in one fell swoop.
- More importantly we don’t want to send our sensitive plaintext data to a third party, regardless of how trustworthy they may seem.
Instead of sending our plaintext data to Cloud KMS, we’re going to generate a unique encryption key every time we write sensitive data to the database. This key is known as a data encryption key (DEK), which will be used to encrypt our data. The DEK is then sent to Cloud KMS to be encrypted, which returns a key-encryption key (known as a KEK). Finally, the KEK is stored side-by-side in the database next to the encrypted data and the DEK is destroyed. The process looks like so:
- Generate a data encryption key (DEK)
- Encrypt the data using secret key encryption
- Send the unique encryption key (DEK) to Cloud KMS for encryption, which returns the KEK
- Store the encrypted data and encrypted key (KEK) side-by-side
- Destroy the generated key (DEK)
When decrypting data the process is reversed:
- Retrieve the encrypted data and encrypted key (KEK) from the database
- Send the KEK to Cloud KMS for decryption, which returns the DEK
- Use the DEK to decrypt our encrypted data
- Destroy the DEK
Cloud KMS and PHP
Like most Google Cloud services, there is a PHP SDK which we can use in our PHP applications The Cloud KMS documentation is quite extensive, but I recommend starting with the Quickstart guide and the Authentication guide, which takes you through all the requirements.
Cloud KMS Requirements
First, you need to create a Google Cloud account and create a new Google Cloud project. Then you enable the Cloud KMS API and install and initialize the Cloud SDK. This step allows to you run the gcloud
command-line tool, which you use to create your Cloud KMS key which is part of a Cloud KMS keyring, and exists in a Cloud KMS location . Finally, you need to create a service account, grant permissions to the service account based on the project and then store the service account credentials in a local credentials file.
While this all seems like a lot of work to set up, the Google Cloud docs are really helpful in guiding you through the steps.
Cloud KMS PHP Implementation
With this in mind, I’ve created a very simple helper class for performing envelope encryption, which uses the Google Cloud KMS SDK. I wouldn’t consider this production-ready code, as it could use some better error handling, but it gives you a place to start. This helper class makes use of the most recent version of the Google Cloud KMS SDK, which is currently at version 1.12.
<?php
namespace MyCloudKmsProject;
use Google\ApiCore\ApiException;
use Google\Cloud\Kms\V1\CryptoKey;
use Google\Cloud\Kms\V1\CryptoKey\CryptoKeyPurpose;
use Google\Cloud\Kms\V1\KeyManagementServiceClient as Kms;
use Google\Cloud\Kms\V1\KeyRing;
class KeyManager{
private $kms;
private $projectId;
private $locationId;
private $locationRef;
private $keyRingId;
private $keyRingRef;
private $cryptoKeyId;
private $cryptoKeyRef;
public function __construct(Kms $kms, KeyRing $keyRing, CryptoKey $cryptoKey, $projectId, $locationId, $keyRingId, $cryptoKeyId)
{
$this->kms = $kms;
$this->projectId = $projectId;
$this->locationId = $locationId;
$this->locationRef = $this->kms::locationName($this->projectId, $this->locationId);
$this->keyRingId = $keyRingId;
$this->keyRingRef = $this->kms::keyRingName($this->projectId, $this->locationId, $this->keyRingId);
$this->cryptoKeyId = $cryptoKeyId;
$this->cryptoKeyRef = $this->kms::cryptoKeyName($this->projectId, $this->locationId, $this->keyRingId, $this->cryptoKeyId);
$this->initializeKeyRing($keyRing);
$this->initializeCryptoKey($cryptoKey);
}
protected function initializeKeyRing(KeyRing $keyRing)
{
try {
$this->kms->getKeyRing($this->keyRingRef);
} catch (ApiException $e) {
if ($e->getStatus() === 'NOT_FOUND') {
$keyRing->setName($this->keyRingRef);
$this->kms->createKeyRing($this->locationRef, $this->keyRingId, $keyRing);
}
}
}
protected function initializeCryptoKey(CryptoKey $cryptoKey)
{
try {
$this->kms->getCryptoKey($this->cryptoKeyRef);
} catch (ApiException $e) {
if ($e->getStatus() === 'NOT_FOUND') {
$cryptoKey->setPurpose(CryptoKeyPurpose::ENCRYPT_DECRYPT);
$this->kms->createCryptoKey($this->keyRingRef, $this->cryptoKeyId, $cryptoKey);
}
}
}
public function encrypt($data)
{
$key = random_bytes(SODIUM_CRYPTO_SECRETBOX_KEYBYTES);
$nonce = random_bytes(SODIUM_CRYPTO_SECRETBOX_NONCEBYTES);
$ciphertext = sodium_crypto_secretbox($data, $nonce, $key);
return [
'data' => base64_encode($nonce . $ciphertext),
'secret' => $this->encryptKey($key),
];
}
public function encryptKey($key)
{
$secret = base64_encode($key);
$response = $this->kms->encrypt(
$this->cryptoKeyRef,
$secret
);
return $response->getCiphertext();
}
public function decryptKey($secret)
{
$response = $this->kms->decrypt(
$this->cryptoKeyRef,
$secret
);
return base64_decode($response->getPlaintext());
}
public function decrypt($secret, $data)
{
$decoded = base64_decode($data);
$key = $this->decryptKey($secret);
$nonce = mb_substr($decoded, 0, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, '8bit');
$ciphertext = mb_substr($decoded, SODIUM_CRYPTO_SECRETBOX_NONCEBYTES, null, '8bit');
return sodium_crypto_secretbox_open($ciphertext, $nonce, $key);
}
}
You’ll notice that the actual encryption and decryption methods are almost identical to the secret key implementation introduced above. The difference however is that we’re now using multiple encryption keys. Let’s see the helper class in action.
KeyManager Usage
To make use of this helper class, you’ll need to start a new project with Composer support, install the google/cloud-kms
package, and make sure to require the Composer autoloader. You will then need to set up the GOOGLE_APPLICATION_CREDENTIALS
constant, using the credentials file you created earlier.
Finally, you need to set up the $projectId
, $location
, $keyRingId
and $cryptoKeyId
variables, which you match the values when you set them up. You can then create a new instance of the KeyManager class, and use it for encryption and decryption.
<?php
putenv("GOOGLE_APPLICATION_CREDENTIALS=credentials.json");
/* Require composer modules */
require __DIR__ . '/vendor/autoload.php';
use Google\Cloud\Kms\V1\KeyManagementServiceClient as Kms;
use Google\Cloud\Kms\V1\CryptoKey;
use Google\Cloud\Kms\V1\KeyRing;
use MyCloudKmsProject\KeyManager;
$projectId = 'project-id-123456';
$location = 'global';
$keyRingId = 'keyring';
$cryptoKeyId = 'key';
$keyManager = new KeyManager(
new Kms(),
new KeyRing(),
new CryptoKey(),
$projectId,
$location,
$keyRingId,
$cryptoKeyId
);
$encrypted = $keyManager->encrypt('My secret text');
var_dump($encrypted);
$unencrypted = $keyManager->decrypt($encrypted['secret'],$encrypted['data']);
var_dump($unencrypted);
If an attacker compromised our system, they would still be able to gain access to our Cloud KMS API implementation, and use that to decrypt any encrypted data they may have copied. If that’s the case, you may be wondering how envelope encryption is any more secure than regular secret key encryption?
The key difference (pun intended) is that the KMS API access can be revoked, thus preventing an attacker from decrypting any data they’ve made off with. With regular secret key encryption where a single local key is compromised, you don’t have that luxury. The attacker has all the time in the world to decrypt your sensitive data, using the local key. Envelope encryption isn’t a flawless solution, but it certainly lowers your risk of a data breach.
WordPress and Sensitive Data
A common question I see in WordPress developer circles is; “How should I encrypt sensitive data like API keys in my plugin or custom theme?”. Usually, these are plugins or themes that need to be able to access some third-party API (eg MailChimp or AWS services), that authenticates using an API access key and sometimes an access key secret. One such example is our own WP Offload Media, which needs access credentials to your offsite storage provider.
Generally, there are two main options available for implementing these access details.
- Store them as plain text with an identifier (usually as a PHP constant) in a credentials file that is not publicly accessible, and require that file from the wp-config.php file.
- Encrypt them, store them in the database, and then decrypt them when you need to make an API call, using one of the encryption methods we’ve discussed above.
The reality is that, whatever you do, if your code can read the secret, so can any motivated attacker. As we’ve discussed, even envelope encryption is not completely secure, and as a plugin developer, you have less control over the encryption methods available to site owners.
In my opinion, it’s better to educate your users on security. Make sure you have documentation in place that they can use to disable third-party API access at the source if a breach ever happens. Make sure that your documentation covers aspects like how to limit read or write capabilities to third-party services. For example, we recently updated our SpinupWP backup storage provider documentation with details on how to restrict API users to specific buckets.
If you work with site owners, teach them to keep WordPress core, plugins, and themes up to date, as well as their PHP version, to prevent vulnerabilities; and to use secure passwords, password managers, and two-factor authentication where possible. If they need to access servers, ensure they are using SSH key authentication and SFTP to manage files.
It’s all well and good changing the locks to your house after someone steals your keys, but it’s better to never have your keys stolen in the first place.
Wrapping Up
Data security and encryption are vast subjects and I’ve covered only a handful of ways to protect sensitive data using PHP. Ultimately though, whatever encryption you implement is still vulnerable to attack, the levels of risk just vary. Knowing the different types does mean you can choose to implement them at different levels of your application code, depending on the sensitivity of your data.
What precautions do you take? Are you using hashing, secret key encryption, or envelope encryption? Let us know in the comments below.