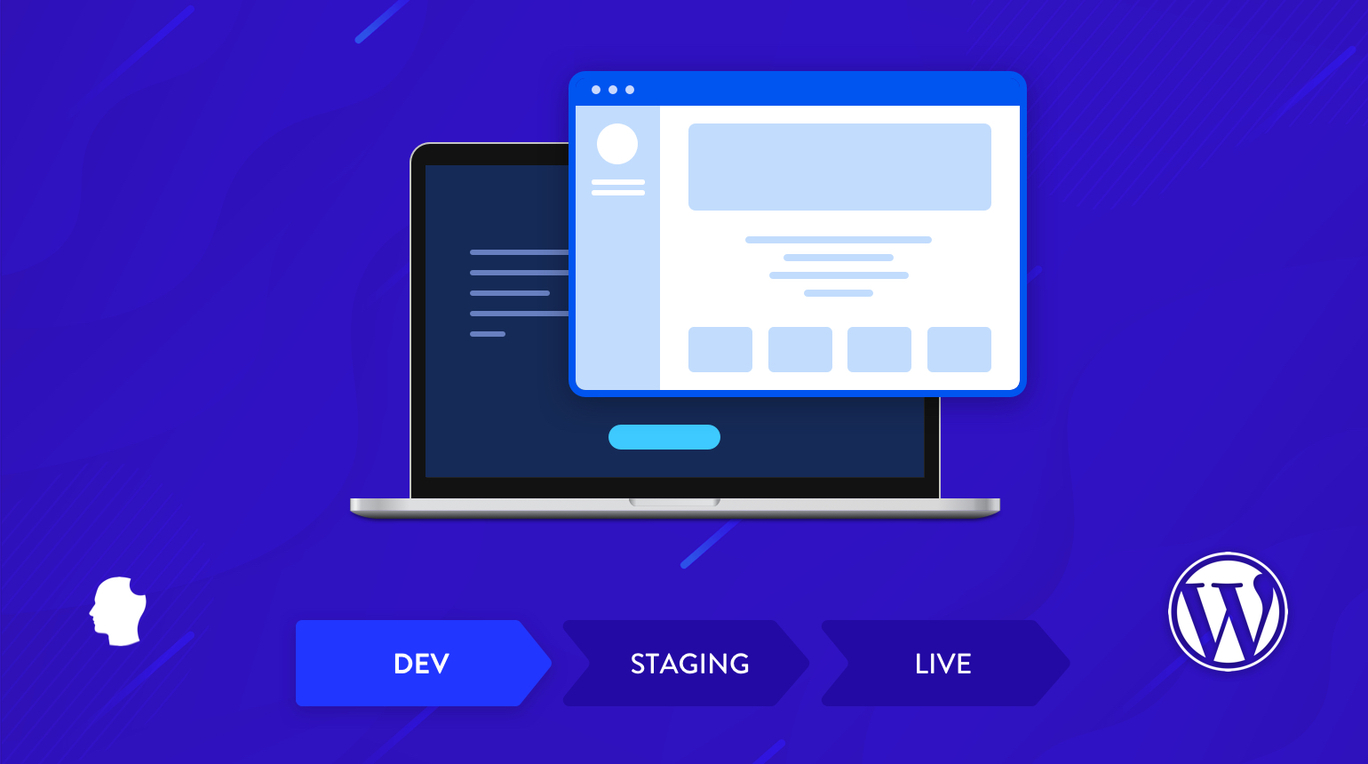
When running a WordPress website it’s best practice to at least run a local copy of the site so you can make changes without running the risk of completely ruining the live site. Running a WordPress dev environment for your sites is crucial to a productive and safe workflow as a developer.
In this post I’ll guide you through various ways to set up the dev environment, install WordPress locally, wrangle the database, debugging and lots in between. There’s a lot to cover so although this post will have a tonne of information, it also features a good collection of links to other articles that dive deeper.
Did you know that WordPress 5.5 introduces a way to set the environment type for a site? In the past developers had to roll their own method of setting the environment. We’ve used our own set of constants before and others have used the WP_ENV
environment variable.
This is necessary to conditionally load code in different environments. For example, instead of sending emails via Mailgun on my development site, I’ll conditionally load a small mu-plugin to deactivate the Mailgun plugin and test emails safely with Mailtrap (I’ll cover Mailtrap in more detail later).
With the WordPress 5.5 release, you can set the environment type using a constant (typically in the wp-config.php file):
define( 'WP_ENVIRONMENT_TYPE', ‘development’ );
You can then use the new wp_get_environment_type()
function in the conditional code:
If ( ‘development’ === wp_get_environment_type() ) {
// run some dev only code
}
Ok, so let’s get into this. The best place to start is with the WordPress dev environment. It’s the first place I start when building a new site and it’s where I spend most of my time developing a site.
WordPress Development Environment
As I mentioned earlier, having a WordPress dev environment is a vital part of a developer’s toolkit, so they can work on sites and make changes without worrying about making mistakes directly on the live site. It’s also a great place to test plugin, theme, and core WordPress updates to check nothing is broken before applying them to the live site. This testing is an important process when updating an ecommerce plugin like WooCoommerce.
However, if you’ve never set up a development environment, it might seem like a daunting task. An environment that can be used for running WordPress sites is made of 3 parts: a web server (generally either nginx or Apache), PHP, and a database management system (typically MySQL).
How to Install a WordPress Dev Environment
There are a large number of software options available to install and run an environment on your computer regardless of your operating system. There are environments around that run on Mac OS, Windows and Linux. I recently asked on Twitter what developers were using for their WordPress dev environment, and the results were interesting. It seems there is a lot of users using the same things, but also a large number of people using bespoke systems:
? Hey #WordPress developers – what are you using for your development environment?
— Iain Poulson (@polevaultweb) August 11, 2020
Please RT for reach ? #webdev
I’ve broken down all the replies and votes in a highly scientific fashion to show the results:
Clearly there are many developers using Local (previously known as Local by Flywheel). Our very own Pete Tasker even wrote about creating a custom addon for Local. A fair few Windows folks are using Laragon (which Matt wrote about ), and a few people are using Devilbox the same as Jonesy. Choosing the right development environment is important, so we’ve spent some time looking into a fair few – we’ve compared MAMP, XAMPP, Local and DesktopServer, taken Docker and Vagrant head to head, and explored how Valet, VVV and Chassis stack up.
I was also interested to see a handful of people saying they use a VPS for their development sites. So instead of having a local development environment on their machine, they have a dev environment on a remote server.
Have a DigitalOcean droplet I use. It's not too much slower than something local and I often need the site at a live domain to accept webhooks and stuff.
— Jason Coleman (@jason_coleman) August 11, 2020
We’ve heard from SpinupWP customers that they use it to do the same, and even our own developers find it sometimes quicker to spin up a new WordPress site on a DigitalOcean server, so they can test features on the latest versions of PHP and MySQL.
Creating a Site
Once you’ve got your WordPress dev environment set up, you’ll need to install WordPress locally. This might be a brand new site you are starting with from scratch, or a site that is a copy of an existing live site that will allow you to make changes to it.
Either way, there are a number of options to create WordPress sites. You could roll your own WordPress site setup script, use WP-CLI, or use Composer. I use the Composer-based setup for SpinupWP as a starting point these days.
Arguably the most important part of a WordPress site is the database. However, the WordPress database doesn’t play nicely with different environments, largely because it stores URLs and file paths in the database rather than keeping everything relative.
This makes moving databases between environments a challenge. But we’ve got you covered.
Moving Databases Between Environments
If you are setting up a site from scratch locally in your dev environment, you would have likely installed the database afresh and when it comes to putting the site live you will need to move that database to the live server.
There are manual ways to move WordPress databases between environments, such as exporting and then importing via PhpMyAdmin. However, the URLs and paths need to be changed in the .sql export file, and doing this with a find and replace will likely break serialized arrays of data.
If you’ve exported and imported the database from one environment to another then you can safely change the URLs and paths using a couple of methods:
- Using the WP-CLI search-replace command
- Using a plugin with a find & replace feature like WP Migrate DB
To take the manual export and import steps out of the equation and save some time, then I recommend using a plugin like WP Migrate DB Pro which will allow you to push and pull your databases between environments.
For example, you’ve just put the finishing touches to a site on your local dev environment and you want to put it live. You can ‘push’ your local database to the live site in a few clicks.
The same goes for when you need to make changes to a live site that has been running for a while. Pull down the latest version of the live database to your local site so you can work on an exact copy which is great for tracking down bugs or working on new features.
Making changes to the database locally and getting them ported back to the live site is a whole different ball game. Short version – try to avoid it. Long version – read how we handle WordPress database merging.
Media Library
We’ve talked about moving databases between environments, but what about the media library? The contents of your site’s uploads folder are an equally important part of a WordPress site. Running a local version of a site without images won’t be helpful when making design or frontend changes.
SFTP is always an option – connect to your live server and copy down the site’s wp-content/uploads directory. Or even using SSH to connect and sync the files with rsync. This is probably the most manual way of doing it. I wrote about different approaches to this problem way back in 2015 and some of the neat tricks like htaccess and add_filter rewrites are still relevant today. But I think my favourite approach is using the Media Files addon for WP Migrate DB Pro, that allows you to push and pull your sites media along with the database.
Local SSL
Back in 2017, Chrome started to redirect all .dev domains to HTTPS , which impacted a large number of developers who used that TLD for their localhost sites. Firefox followed suit in 2018. Developers suddenly needed to create SSL certificates for their local sites.
But that’s not the only reason to have a certificate locally. Replicating your production site as closely as possible is a good idea to make sure you can fully troubleshoot issues. For example, mixed content warnings won’t be evident until the site is deployed with SSL. Another reason for local SSL is integration with third-party services, like webhooks or oAuth integrations that require HTTPS.
There are various ways to get a local SSL certificate, and it can depend on what you choose to use for your dev environment. If you are using Valet, then you can use the CLI command valet secure
to turn on SSL for the site. Local and MAMP Pro have the ability to generate and use a certificate for the host, right in the UI. If you want to get a bit more hands on, then check out our guide to generating SSL certificates and creating a certificate authority.
Debugging
In my opinion, one of the biggest benefits of having a WordPress dev environment for a site is to make it easier to debug problems. If you’ve ever tried to solve an issue on a live site armed just with FTP access and error_log
lines, you will know just how tedious and hairy it can be.
The essential tool in a WordPress developers toolbox for debugging is WP_DEBUG. Turning this on in your local wp-config.php
file, along with WP_DEBUG_LOG will write all PHP errors, notices and warnings to the site’s wp-content/debug.log
file:
define('WP_DEBUG', true);
define('WP_DEBUG_LOG', true);
define('WP_DEBUG_DISPLAY', false);
Although WP_DEBUG is turned off by default, and typically it’s not turned on for live sites, I actually recommend using the same code on production sites to catch errors that happen for real, and use a service like Papertrail to give you better visibility of the logs.
Having a site running locally, with a fresh sync of the live database, and WP_DEBUG on, makes the debugging process a lot easier – and safer! Coupled with using a debugging tool like Xdebug, you’re much better equipped to recreate and track down bugs.
Xdebug allows you to set breakpoints on specific lines of code, so that when those lines of code are executed by a request, the execution is paused and you get an insight into all the variables, and globals, helping you figure out what isn’t set, what array is actually an object, or whatever piece of code is broken.
Other Tips
When running a WordPress development environment there are a few other things that you should consider, that will improve your workflow.
For live sites, I recommend using a transactional email service plugin to handle sending emails, but you don’t want to be sending emails from your local site through this service. Aside from an increase in cost by adding to your usage, you don’t want to accidentally send emails to customers if an email is accidentally sent from your local site.
I’m a big fan of Mailtrap which provides a safe email environment for your dev and testing sites. It has a free plan for personal projects, and allows you to send your emails via their SMTP server to your own sandboxed email inbox.
In all my sites I add this mu-plugin to disable the transactional email plugin and tell WordPress to send emails via Mailtrap when the site is running on a non-production environment:
<?php
If ( ‘production’ === wp_get_environment_type() ) {
return;
}
add_filter( 'option_active_plugins', function ( $plugins ) {
if ( ! is_array( $plugins ) || empty( $plugins ) ) {
return $plugins;
}
foreach ( $plugins as $key => $plugin ) {
if ( 'mailgun/mailgun.php' === $plugin ) {
unset( $plugins[ $key ] );
}
}
return $plugins;
} );
add_action( 'phpmailer_init', 'mailtrap' );
function mailtrap( $phpmailer ) {
$phpmailer->isSMTP();
$phpmailer->Host = 'smtp.mailtrap.io';
$phpmailer->SMTPAuth = true;
$phpmailer->Port = 2525;
$phpmailer->Username = '{mailtrap_username}';
$phpmailer->Password = '{mailtrap_password}';
}
Plugins
There are a few WordPress plugins I will always install on my local WordPress sites:
- Query Monitor – my go-to plugin for debugging slow WordPress sites, finding slow or duplicate SQL queries, and investigating bottlenecks in code.
- Debug Bar – great for getting an insight to requests, cache and other WordPress site information right from the admin bar.
- WP Crontol – gives you insights into the WordPress cron scheduling system, so you can understand issues with scheduled events.
Because my sites are managed with Composer (WordPress core and plugins), I install them as dev dependencies so they don’t make it to the live site. For example:
composer require --dev wpackagist-plugin/query-monitor
I then enable the “Do not migrate the ‘active_plugins’” setting in my WP Migrate DB Pro profiles, so that whenever I pull the live site database back to my local, these development plugins won’t be deactivated.
WP-CLI
WP-CLI is an extremely useful tool. It makes life easier by allowing you to manage WordPress sites on the command line. There are a number of commands that can come in handy in your WordPress dev environment:
- wp core install – install WordPress in one command
- wp site empty – truncates the post, terms and comments tables, but leaving the options table intact
- wp db reset – drops all tables in the database, allowing you to do a fresh install
- wp server – launch PHP’s built-in web server for a specific WordPress installation.
Git
I speak to a lot of freelancers at the WordPress meetup I run. Many believe they don’t need to learn and use Git because they are working on their own, instead of in a team. They see version control as something only useful or required for collaboration. However, the benefits of using git for your sites apply to solo developers too.
Keeping your site project version controlled and stored on a distributed system like GitHub or Bitbucket means you get backups of your code. Deployed some changes to the live site that you need to rollback? If the site only lives in your file system, then that’s a lot of CMD+Z’ing to undo your changes. With code version-controlled with Git, you can rollback your recent commits or easily see a diff of recent changes for your files.
Take a look at some other resources WordPress recommends for setting up a development environment.
Wrapping Up
Hopefully this has been a comprehensive guide to WordPress development environments and running WordPress locally. I’ll be working on guides to the best practices for running both a staging environment and a production environment – so keep an eye out for those.
What’s your favorite development environment? Any tips and tricks for local development I’ve missed? Let me know in the comments below.